Comment Script
A Revolutionary Comment-Driven Programming Language
A few years ago I wrote a Blog Post explaining why I thought using comment in code could be a bad practice. I was wrong.
Imagine a future where anyone who can write in English can build robust, production-quality software. With CommentScript, we are redefining software development by decoupling programming logic from conventional syntax. Instead of learning the intricacies of a specific programming language, developers write plain English comments, which are then translated into fully functional code by our advanced Comment Compiler.
How CommentScript Works
In CommentScript, your entire application is expressed as natural language comments. The Comment Compiler intelligently parses these comments, generating the underlying code automatically. Additionally, it injects debug statements based on your inline directives, allowing for streamlined debugging and effortless tracking of code behaviour.
Consider the following CommentScript example:
/**
* Function that adds two numbers a and b as argument
* and returns the sum.
*
* The function should only accept positive number and
* should throw an exception for negative number.
*/
add_number.cs
The CommentScript Compiler will process this comment and generate automatically the following TypeScript (or any other language) code:
function addPositiveNumbers(a: number, b: number): number {
if (a < 0 || b < 0) {
throw new Error("Both numbers must be positive.");
}
const sum = a + b;
return sum;
}
No need to learn programming, all you need to learn is to write a good comment. And if your company decide to switch to another programming language all you need is to process all your comments source file with a new programming language.
Here in Rust:
fn add_positive_numbers(a: i32, b: i32) -> i32 {
if a < 0 || b < 0 {
panic!("Both numbers must be positive.");
}
let sum = a + b;
sum
}
And here in Erlang:
-module(math_utils).
-export([add_positive_numbers/2]).
add_positive_numbers(A, B) when A < 0; B < 0 ->
error({invalid_input, "Both numbers must be positive."});
add_positive_numbers(A, B) ->
Sum = A + B,
Sum.
How this work
CommentScript work in a two steps process. First it read all the Comments files and takes a target programming language as an input.
The first step is to generate all the source files from the comments files, and then call the compiler to create the final working program.
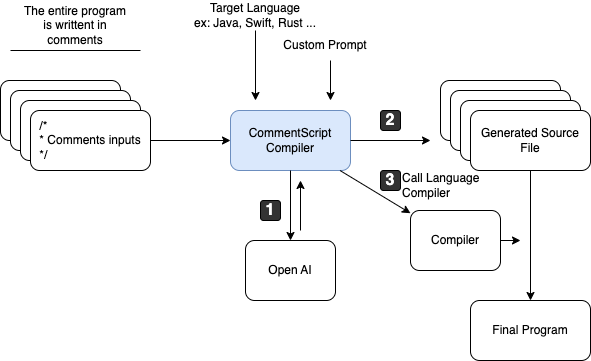
Since CommentScript didn't exist we actually had to write some complex and difficult code to implement our comment compiler. We did it knowing this was the last time we would be writing code.
And today we have the pleasure to announce that we are Open Sourcing CommentScript.
Advanced Debugging Capabilities
CommentScript includes built-in debugging support. By simply annotating your code with debug directives, the Comment Compiler automatically inserts the necessary logging statements. This ensures that every critical operation is traceable, greatly simplifying the debugging process. For example:
/**
* Function that return true if the move for a Knight is valid for
* a game of chess.
*
* @debug Log both the inputs and the resulting product.
*/
The compiler transforms these directives into executable code with integrated logging, allowing developers to monitor the execution flow and quickly identify any issues. Here is Java:
public class ChessValidator {
private static final Logger logger = Logger.getLogger(ChessValidator.class.getName());
public static boolean isValidKnightMove(int startX, int startY, int endX, int endY) {
logger.info(String.format("Checking move from (%d, %d) to (%d, %d)", startX, startY, endX, endY));
int dx = Math.abs(startX - endX);
int dy = Math.abs(startY - endY);
boolean isValid = (dx == 2 && dy == 1) || (dx == 1 && dy == 2);
if (isValid) {
logger.info("Move is valid.");
} else {
logger.warning("Move is invalid.");
}
return isValid;
}
}
Notice how concise is the CommentScript source compare to the actual converted code.
Fast Integration Scripting Header
When working with Comment we face one difficulty on dependency between them. Let say we are implementing a game of chess and we want to implement a Board, a Piece and a Knight, when generating the actual code from comment we need to provide context between each other. This is something you would normally do in standard programming language with an Import.
In CommentScript we introduce the Fast Integration Scripting Header to link multiple source files with each other. What this does under the hood is to include the imported dependency when building the prompt used when generating the source file from comment. Here is action:
/**
* @fish: piece.cs
*
* Object representing a chess board using a grid of 8 by 8 cells.
* Each cells can be empty or contains a @Piece.
* When constructing a new Board initialise the grid with all
* the standard piece used in the game of Chess in their correct
* position and color.
*/
board.cs
/**
* @fish: board.cs
*
* Object representing any Piece for the game of chess.
* A Piece has a color black or white.
* A Piece can move and each implementation of a piece have
* it's own way of moving
*/
piece.cs
/**
* @fish: board.cs
* @fish: piece.cs
*
* Object representing an Knight of the game of chess.
* When moving the piece in the Board validate that the move
* is valid considering how the knight move in chess (L shape)
* Also consider the Board and validate that the move is valid
* considering other piece.
*/
knight.cs
The Benefits of CommentScript
Universal Accessibility
- Inclusive Development: With CommentScript, anyone with proficiency in English can contribute to building sophisticated applications.
- Lowered Learning Curve: Developers no longer need to invest extensive time in mastering language-specific syntax, leading to faster onboarding and more efficient collaboration.
Decoupled and Portable Code
- Language-Agnostic Codebase: The natural language comments source file can be compiled into various target languages, making your code highly portable and adaptable to future technologies.
- Future-Proofing: As programming paradigms evolve, CommentScript offers a stable intermediary representation that can be transformed into modern code constructs as needed.
Streamlined Debugging
- Automated Log Generation: By embedding debug instructions directly in your comments, CommentScript eliminates the manual effort of adding debug code, thereby reducing errors and improving code maintainability.
- Enhanced Traceability: With automated debug logs, developers can trace application behavior in real time, ensuring rapid identification and resolution of issues.
A Paradigm Shift in Software Development
CommentScript represents a significant leap forward in software development. By transitioning from traditional coding paradigms to a comment-driven approach, we are making programming more accessible, reducing the complexity of syntax, and empowering a broader range of contributors to bring their ideas to life.
Our organization is already adopting CommentScript across our entire codebase, witnessing improvements in productivity, code clarity, and team collaboration. We believe this innovative approach is the future of software engineering—one where natural language and advanced compilation techniques converge to create more intuitive, maintainable, and portable code.
We have quite a large code base at Michelin and are currently developing a code converter that take all the source files of our application to convert it in CommentScript format (which is already part of our Open Source project).
Welcome to the new era of programming with CommentScript.
Applying "CommentScript" to Blogging
Instead of manually writing a full blog post, authors could annotate their thoughts as structured comments and let the AI expand them into a polished article.
Example: Comment-Driven Blogging
Imagine writing a blog post with just high-level comments:
// Title: The Future of AI-Driven Software Development as an April Fool
// Introduction: Explain how AI is automating coding and software engineering 🐟
// Section 1: Current AI-assisted coding tools (Copilot, ChatGPT, etc.) 🐠
// Section 2: How AI-generated code is improving productivity 🐡
// Section 3: Challenges and risks (bugs, security, reliance on AI) 🎣
// Conclusion: How "All Comment" could revolutionize both programming and blogging
With an AI-enhanced pre-compiler for text, these comments would be converted into a well-structured, coherent blog post.